
In the world of business, partnerships are often the key to success. Whether it’s collaborating with nearby businesses for mutual benefit or reaching out to potential allies in the market, the process of identifying and connecting with partners can be both time-consuming and prone to errors. However, with the advent of technology and the availability of powerful APIs, this task can now be streamlined and automated, saving valuable time and resources.
This article serves as a companion to our YouTube short.
Overview
At Endalon, we recently embarked on a mission to compile a comprehensive list of nearby businesses for potential partnerships. At first this involved manually scouring through Google Maps search results, extracting relevant information, and painstakingly inputting it into spreadsheets. Needless to say this method was not only tedious but also susceptible to mistakes, particularly when dealing with phone numbers and emails in large volumes.
About Google’s APIs
Fortunately we are programmers and remembered the existence of one gem: Google’s API, specifically Google’s Places API. This powerful API provides access to a wealth of business information, including contact details, addresses, and customer reviews, all with just a few lines of code. By leveraging this API, we were able to automate the process of gathering business data, significantly reducing the time and effort required (if you think getting a program to work isn’t a lot of time and effort).
Implementing the Google Places API into our workflow was surprisingly straightforward. Armed with a Google API, to which Google provides a generous allowance of $200 free usage every month, we were able to access and retrieve the data we needed.
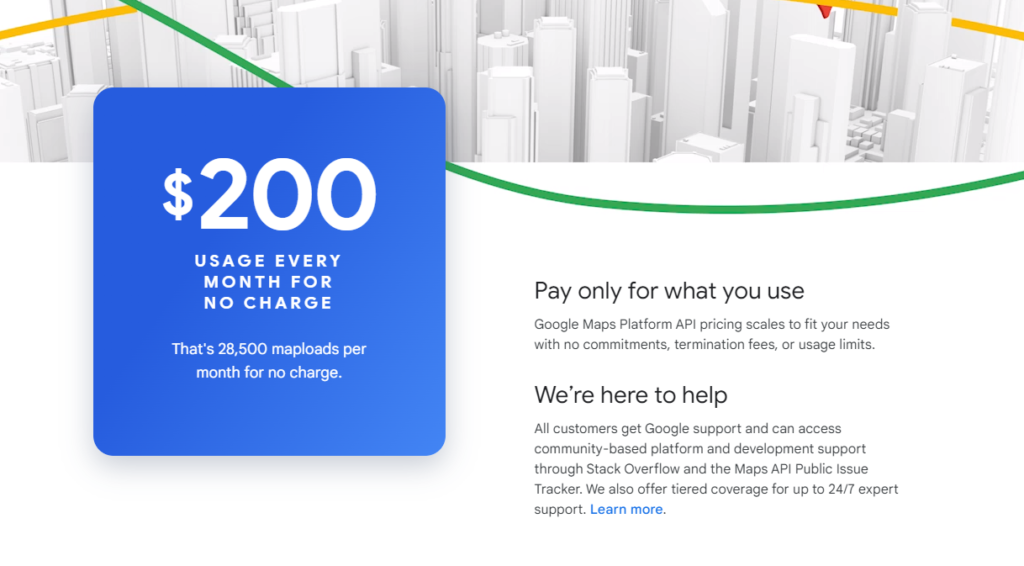
The beauty of the Google Places API lies in its versatility. Depending on your preferences and technical capabilities, there are various ways to integrate it into your workflow. For instance, we opted to develop a custom program using C# and the Google API Framework. This allowed us to create a tailored solution that seamlessly converted the retrieved data into a .CSV file, compatible with Excel—a popular choice for managing business information.
Implementation
To illustrate how straightforward the implementation process can be for programmers using C#. For convenience we have leveraged this Google API Nuget Package to handle the API requests (big thanks to who made this!) Here’s a basic snippet of code we wrote to set up our application:
using GoogleApi;
using GoogleApi.Entities.Common;
using GoogleApi.Entities.Common.Enums;
using GoogleApi.Entities.Places.Common.Enums;
using GoogleApi.Entities.Places.Details.Request;
using GoogleApi.Entities.Places.Search.Common.Enums;
using GoogleApi.Entities.Places.Search.NearBy.Request;
// Assign an API key
var apiKey = "{YOUR_API_KEY_HERE}";
// Create a file to write the csv data to
await using var writer = new StreamWriter("results.csv");
// We use quotation marks here to ensure that the values aren't accidentally translated into separate fields
writer.WriteLine("\"Name\",\"Type\",\"FormattedAddress\",\"Website\",\"FormattedPhoneNumber\"");
// Create a request to find all electronic stores close to Maastricht in a radius of 5KM
var nearbyPlacesRequest = new PlacesNearBySearchRequest
{
Location = new Coordinate(50.851817192973236, 5.692010360004194),
Radius = 5 * 1000,
Type = SearchPlaceType.ElectronicsStore,
Key = apiKey
};
// Send the request and store all the nearby place
var allNearbyPlaces = await GooglePlaces.Search.NearBySearch.QueryAsync(nearbyPlacesRequest);
// For each place we found we need to make another request to get the information
foreach (var business in allNearbyPlaces.Results)
{
// Create a "GetDetailsRequest" and specify the details we want
var placeDetailsRequest = new PlacesDetailsRequest
{
Key = apiKey,
PlaceId = business.PlaceId,
Language = Language.Dutch,
Fields = FieldTypes.Website | FieldTypes.Formatted_Address | FieldTypes.Name |
FieldTypes.Formatted_Phone_Number
};
// Send the request and store the details
var placeDetails = await GooglePlaces.Details.QueryAsync(placeDetailsRequest);
// Write all of the details in order to the CSV file
writer.WriteLine(
$"\"{placeDetails.Result.Name}\",\"{nearbyPlacesRequest.Type}\",\"{placeDetails.Result.FormattedAddress}\",\"{placeDetails.Result.Website}\",\"{placeDetails.Result.FormattedPhoneNumber}\"");
}
Conclusion
In this article we have wanted to show you our process of utilizing the Google API to automate our work.
SUPPORT US
Did you find this article helpful? Join our Patreon community to unlock exclusive rewards. Your support fuels the creation of valuable resources like this one and helps us continue to pursue our goals. Together, we can continue our mission to help individuals realize their goals and projects through a combination of tutorials, online courses, and entertaining videos.
Do you want to talk with like-minded people? Join our Discord.
Have any questions? Feel free to email us at [email protected].
Leave a Reply